Link your Form with Google Form for Responses
Forms are a core element of any modern website from a company using it to get feedback to an early startup getting reviews. The form is expected to blend in your website with the design. You won’t like to add something off-styled in your website.
Google Form
Google forms give you the power to create very complex looking forms within minutes and it also helps you in collecting data with Google Sheets. But when it comes to adding a form to your website, embedding google form might not be a good idea.
- In this blog, we would work out on building a form for our website
- Using Google form for form submission and data storage with google sheets
Create a Custom Form
Let’s start by creating a form where we would ask for the name and email of a person. Later, we will look to integrate it with our google form.
<form>
<input class="input" type="text" placeholder="Name" />
<input class="input" type="email" placeholder="Email" />
<button class="button" type="submit" >
Submit
</button>
</form>
The above code creates a simple form with two inputs name and email, and a submit button.
You will need to style the components as per your design while working in a project.
Creating Google Form
- Create a new google form
- Add Name input
- Add Email input
[Don’t worry about the capitalization of characters in the name of inputs]
- Copy your google form Id, you will find it in the URL of your google form.
Now, let's update our custom form.
- Add action attribute in form tag with the following URL.
https://docs.google.com/forms/d/{YOUR_FORM_ID_HERE}/formResponse
- Add POST in method attribute in form tag
Now your form code would look like this
<form
method="POST" action="https://docs.google.com/forms/d/{YOUR_FORM_ID_HERE}/formResponse"
>
<input class="input" type="text" placeholder="Name" />
<input class="input" type="email" placeholder="Email" />
<button class="button" type="submit" >
Submit
</button>
</form>
Getting Form Field Ids
To get the field ids, inspect the “name” attribute of each input tag. Let’s see how to do this.
- Inspect the input element.
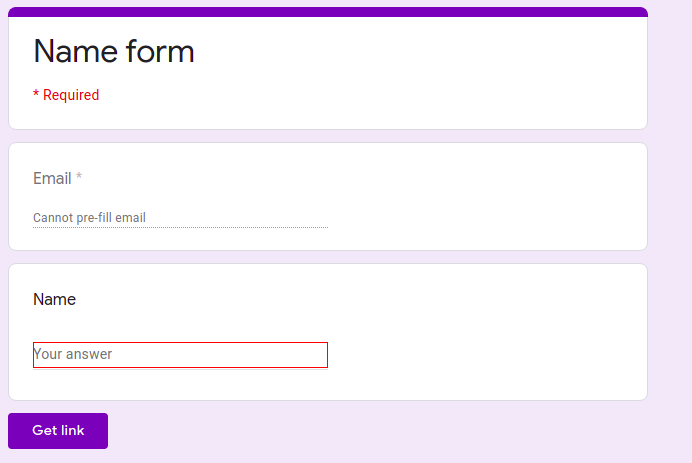
2. Inspect the name attribute in the input element.

Inspect and get the name attribute in the prefilled link. Due to some google form changes, the same is not visible now in form editing or form submission pages. For more about this, refer Update [27 Jun 2021] in bottom of this blog.
Or run the following script in the console on the form page. [Run the script while in preview mode or while in the pre-filled link of your form. ]
function loop(e){
if(e.children)
for(let i=0;i<e.children.length;i++){
let c = e.children[i], n = c.getAttribute('name');
if(n) console.log(`${c.getAttribute('aria-label')}: ${n}`);
loop(e.children[i]);
}
}; loop(document.body);
Should print something similar to this in your console
name: entry.748645480
email: entry.919588971
Now add the name attribute to the respective input tag in your custom form.
Now your code would look like
<form
method="POST" action="https://docs.google.com/forms/d/{YOUR_FORM_ID_HERE}/formResponse"
>
<input
class="input"
type="text"
name="entry.748645480"
placeholder="Name"
/>
<input
class="input"
type="email"
name="entry.919588971"
placeholder="Email"
/>
<button class="button" type="submit" >
Submit
</button>
</form>
- Now fill in the form and click on submit your response would be recorded.
- To verify, check the response of your form or view the google sheets with responses from the form.
- After Clicking on Submit, a new webpage opens up of google form but isn’t it bad to get a user off your website and landing up on another website.
- You can add a target attribute to your form to make your form to open and submit the response in a new tab. This way a new tab will open and your website wouldn’t be closed.
Smarter Work
Although form handling via google form is pretty cool, you won’t like to show any webpage or form handling or response from google while creating your own form. You don’t want your user to see that your form is actually being handled by Google.
So we need to change our approach of form submission to make this happen. Rather than handling form submission via form tag, we would make an HTTP request for form submission.
We would make a request like this:
https://docs.google.com/forms/d/12WFwgQkmjGRcWS52-prj5Lo7dthvFGiR6mJheXlTMuk/formResponse?entry.1859390625=amankumar&entry.960670075=mymail
- Divert your form submission to a function, create a new function handleSubmit to handle your submit and pass it to the onSubmit attribute in the form tag.
<form
onSubmit="this.handleSubmit"
>
I am giving the idea while making the request in ReactJS you can work out the same via any method.
- handleSubmit function
We would use the React States to get the input data. You can keep it your way to get the data.
The following is some snippets of code from your form class inside ReactJS
//Inside Class
myRequest = (url) => {
let response;
try {
response = axios.post(url,null,null)
} catch (e) {
response = e;
}
console.log(response)
}handleChange = (e) => {
this.setState({ [e.target.name]: e.target.value })
}handleSubmit = (e) => {
const data = {
...this.state
}
const id = "12WFwgQkmjGRcWS52-prj5Lo7dthvFGiR6mJheXlTMuk" //YOUR FORM ID
e.preventDefault()
const formUrl = "https://docs.google.com/forms/d/"+ id +"/formResponse";
const queryString = require('query-string')
const q = queryString.stringifyUrl({
url: formUrl,
query: data
})
this.myRequest(q)
}//Render and return the following element from class
<form
onSubmit={this.handleSubmit}
>
<input
class="input"
type="text"
name="entry.748645480"
placeholder="Name"
onChange={this.handleChange}
/>
<input
class="input"
type="email"
name="entry.919588971"
placeholder="Email"
onChange={this.handleChange}
/>
<button class="button" type="submit" >
Submit
</button>
</form>
TroubleShooting
- Check for a mistake in form id
- Check that the field Ids are correct
- If null responses are being stored, check for your data handling
Observation:- While handling the form submission via HTTP request error is being returned from the server, although data is being successfully stored.
Update
[27 Jun 2021]
There have been quite big changes in the google form.
- You can’t find the name attribute of an input element in the page where form is being edited. Neither anyone who has a link to submit the form can find the attribute. If you try inspecting on these pages. Here is how a input element would look like. NOTICE NO NAME ATTRIBUTE.
<input type="text" class="quantumWizTextinputPaperinputInput exportInput" jsname="YPqjbf" autocomplete="off" tabindex="0" aria-labelledby="i5" aria-describedby="i6 i7" dir="auto" data-initial-dir="auto" data-initial-value="">
Solution
Thankfully, there is a simple workaround for this, I found it here. Just click on the hamburger button and get a prefilled link to the google form. You can get the name attributes of the form elements at the prefilled link.
I did the same and got the name attribute.
<input type="text" class="quantumWizTextinputPaperinputInput exportInput" jsname="YPqjbf" autocomplete="off" tabindex="0" aria-label="Name" aria-describedby="i.desc.1379593100 i.err.1379593100" name="entry.1732014374" value="" dir="auto" data-initial-dir="auto" data-initial-value="">
Hope this helps!! 😄